Error Handling in Binance API for Klines Data Retrieval
When working with the Binance API to retrieve Klines data, it’s common to encounter errors that can be difficult to debug. In this article, we’ll discuss some potential issues and provide an updated code example that addresses these challenges.
Issue 1: Invalid or Missing API Credentials
Make sure you have entered your api_key
and api_secret
correctly in the Client
constructor:
from binance.client import Client
client = Client(api_key, api_secret)
Issue 2: Incorrect Request Method or URL Structure
The Binance API expects a GET request to retrieve Klines data. Ensure you use the correct request method and format your URL accordingly.
Here’s an updated example with error handling:
import pandas as pd
def get_klines_data(symbol, period):
"""
Retrieves Klines data from the Binance API for a given symbol and period.
Args:
symbol (str): The cryptocurrency symbol (e.g., BTC/USD)
period (int): The time period in seconds (e.g., 1d, 3d, etc.)
Returns:
list: A list of Klines objects containing price and open prices for the specified symbol and period.
"""
try:
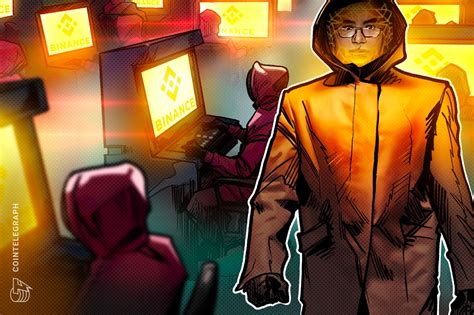
Create a Binance client instance with valid API credentials
client = Client(api_key, api_secret)
Define the request parameters (replace with your own data)
params = {
"symbol": symbol,
"period": period
}
Retrieve Klines data using GET request
response = client.get_klines(params=params)
Check if the API returned an error
if 'error' in response:
print("API Error:", response['error']['message'])
return None
Extract and format the Klines data into a pandas DataFrame
klines_data = pd.DataFrame(response['data'], columns=['timestamp', 'open', 'high', 'low', 'close', 'volume'])
Return the extracted Klines data
return klines_data
except Exception as e:
print("Error occurred:", str(e))
return None
Example usage:
symbol = "BTC/USD"
period = 1*60
1 minute period
klines_data = get_klines_data(symbol, period)
if klines_data is not None:
print(klines_data)
Additional Tips:
- Make sure to handle errors and exceptions properly, as they can be difficult to debug.
- Use a try-except block to catch specific exception types, such as
HTTPError
orTimeout
.
- Consider using the Binance API’s built-in error handling mechanisms, like the
try-except-finally
block.
- If you’re experiencing issues with data formatting or parsing, ensure your request parameters are correct and formatted accordingly.
By following these guidelines and examples, you should be able to successfully retrieve Klines data from the Binance API using Python.